If you've been learning to program for even a short amount of time, chances are good that you have come across the term API. So, what is it?
A quick google search returns the following top result:
"API is the acronym for Application Programming Interface, which is a software intermediary that allows two applications to talk to each other."
At a high level, an API defines a set of protocols (rules) allowing a computer (server) to communicate with another computer (server). An API defines the rules of this transaction, including what data is sent and received, how that information is transmitted between computers, and who is authorized to access or interact with the information.
HTTP is not REST
HTTP (Hypertext Transfer Protocol) is a way to send information between two computers and is a protocol that REST APIs typically utilize. Most websites today are built using HTTPS (HTTP Secure), an enhanced version of HTTP that transfers data more securely. HTTP(S) follows the client-server model to communicate via the request-response cycle, where a client opens a connection to make a request and waits until it receives a response from the server. The request-response cycle is the information flow between a client and a server when the client needs access to a resource (e.g., document, photo, etc.) provided by a server. The server does not hold on to any data between requests, making HTTP a stateless protocol.
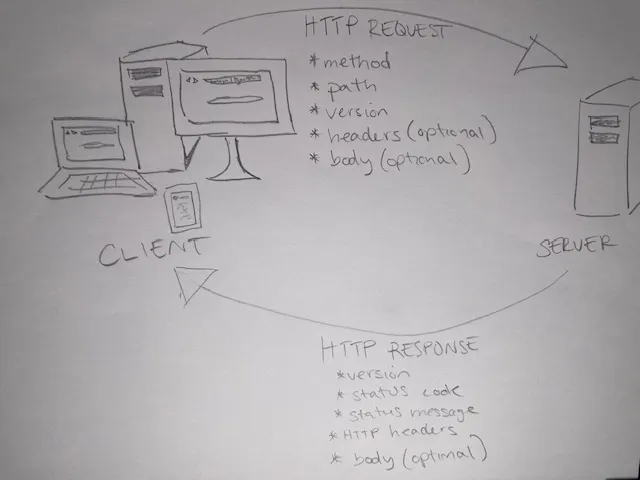
The client can access and modify these resources through a request. After a request is received, the server sends a response back with the status of the request.
Requests and Responses
The structure of a request and a response is relatively similar.
Request
A suitably formatted request contains the following:
- a request line
- HTTP headers or header fields
- a message body (if needed)
Request line - includes the path to the target resource; a method; the version of HTTP
- path - the target resource or resources for the request
- method - a verb that describes an action to be performed on a resource:
- POST = Create a new resource
- GET = Retrieve a resource or resources
- PUT = Update an existing resource
- DELETE = Delete an existing resource
- version - indicates what version (and what structure) to use in the response
HTTP headers - pass additional information/context (e.g., preferred language, what types of data content can be understood by the client, etc.). In HTTP/1.1, the HOST header is mandatory.
Message body - required to add a new resource or update an existing resource
GET request
GET /api/breeds/image/random HTTP/2
Host: www.dog.ceo
You can test requests with the library curl via the computer terminal, a tool like Postman, or an IDE.
curl https://dog.ceo/api/breeds/image/random
Note: curl only requires `curl` followed by the target URL to process a GET request.
Response
A suitably formatted response contains the following:
- A status line
- HTTP headers or header fields
- message body
Status line - includes the version of HTTP (typically 1.1); a status code; a status message
- version - indicates the version and structure used in the response
- status code - an indication of the success or failure of a request.
- 200-299 = successful responses
- 300-399 = redirection messages
- 400-499 = client error responses
- 500-599 = server error responses
- status message - human-readable text that summarizes the status code
HTTP headers - additional information/context (e.g., additional info about the response and the server, how to remedy an unsuccessful request, etc.)
Response body (optional) - information/data in the format specified by the original request
GET request (bad path)
curl https://dog.ceo/api/breeds
Making a request returns the following:
{
"status":"error",
"message":"No route found for \"GET \/api\/breeds\/\" with code: 0",
"code": 404
}
What makes an API RESTful?
REST is an acronym that stands for REpresentational State Transfer. When a client requests a resource using a REST API, a representation of the resource is returned from the server. The representation is the current state of the resource. Like the HTTP protocol, a REST API is stateless since it returns any pertinent information about the resource to the client and does not hold on to any information about previous requests.
REST is not a protocol like HTTP, though it is common to see the two terms used interchangeably since the HTTP protocol exhibits many features of a RESTful system. REST is an architectural style with specific constraints. Given this, RESTful APIs will often differ since constraints aren't hard and fast rules and implementation details are left open to interpretation.
REST Constraints
Several constraints should be honored for an API to be considered RESTful:
Separate client and server
The client and the server are separate, allowing each to evolve independently. The client's job is to request services. The server's job is to respond to requests and provide the requested services. In this model, multiple different clients can make requests.
Stateless
No state from the client should be stored on the server, and any information about the client's session should be stored at the user level with the client. The server should not hold anything from a single client request, and every request sent to the server (from one or multiple devices) does not know about any other requests. This approach allows for less complexity and makes it easier to scale.
Cache
REST APIs should allow for caching (storing) data at the application level - making this information available to all users. Caching reduces unnecessary repeated requests to the server, improving client-side performance because the data is delivered faster. A REST API will categorize response data as either being cacheable or non-cacheable.
Hypermedia as the engine of application state (HATEOAS)
Many REST implementations are not HATEOAS-compliant due to the complexity of implementing this as part of the architecture. This constraint states that dynamic hypermedia (links to different parts of the API) should be part of the server response. Hypermedia allows for self-descriptive responses that communicate what additional, related requests are possible through the API.
GET /random HTTP/2
Host: api.publicapis.org
{
"count":1,
"entries":[{
"API":"Correios",
"Description":"Integration to provide information and prepare shipments using Correio's services",
"Auth":"apiKey",
"HTTPS":true,
"Cors":"unknown",
"Link":"https://cws.correios.com.br/ajuda",
"Category":"Tracking"
}]
}
Uniform interface
This is the most crucial constraint for making an API RESTful. REST APIs should have a uniform interface that establishes a generalized contract between the client and server so that information can be exchanged in a standardized way. There are four constraints to uniform interfaces:
Identification of resources
URIs identify the unique name and address of a resource. Once a resource identifier has been created, it should always exist to ensure stability.
URI
http://www.example.org/owners
Manipulations of resources via representations
REST APIs should not return the literal resource from the database. Instead, the server should send a representation of the resource (and the current state and any metadata/additional data) back in the response. That representation could be JSON, XML, HTML, etc.
Request & JSON Response
GET owners/123 HTTP/2
Host: www.example.org
{
"id": "123",
"name": "Jane Doe"
}
Self-descriptive messages
Every representation sent back from the server should carry enough descriptive data and metadata in the response headers to process the response. Responses include information such as the media type, the date it was last modified, whether it is cacheable, etc.
Request & Response headers
GET owners/123 HTTP/2
Host: www.example.org
< HTTP/2 404
< date: Wed, 20 Jul 2022 17:12:42 GMT
< content-type: application/json
< content-length: 95
< x-powered-by: PHP/7.3.31
< cache-control: no-cache, private
< access-control-allow-origin: *
...
Layered System
In a REST architecture, a response or request may interact with multiple servers during the request-response cycle. These servers may deal with caching, load balancing, or other functions. Layered systems allow for better security since the client should not know if they are interacting with the final layer. These systems also create less complexity due to the separation of concerns.
Note: Code on Demand is an optional REST constraint that sends code in the response that the client can execute. You can read more about this optional constraint here.
Conclusion
Although HTTP has many features of a RESTful system, HTTP and REST are different. Following REST constraints and conventions when building an API is critical to take advantage of the benefits (flexibility, less complexity, performance, etc.) of RESTful systems.